In the world of web development, two well-known technologies developed by Google for dynamic web applications are AngularJS and Angular. Although they share a similar name, their architecture, functionality, and use cases differ significantly.
- AngularJS is a JavaScript-based framework designed to build simple, single-page applications (SPAs) with features like two-way data binding and dependency injection.
- Angular, on the other hand, is a TypeScript-based platform built for scalable and complex applications with a modern, modular architecture.
This blog provides a detailed comparison of AngularJS vs. Angular, covering their features, advantages, disadvantages, and use cases, helping you determine which one is best for your project.
What is AngularJS?
AngularJS is a JavaScript based front-end framework designed to develop simple dynamic single-page web applications (SPAs). It offers innovative features like two-way data binding, Dependency Injection (DI), Model-View-Controller(MVC) architecture and many more.
Key Features of AngularJS
1. Two-way Data Binding:
Synchronizes the UI and the model in real time, this means that any modifications made to the user interface are automatically mirrored in the model, and vice versa.
For example, if the user modifies the value in any input field, the AngularJS property will be updated accordingly.
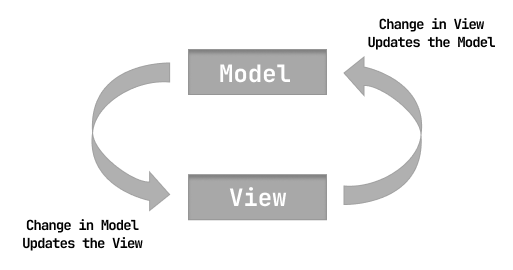
2. Directives:
Instructs the HTML compiler of AngularJS to add a particular behaviour to that DOM element.
Types of Directives :
- Built-in directives (e.g., ng-app, ng-controller, ng-repeat).
- Custom Directives (developers can create their own reusable components).
3. Dependency Injection:
It is a software design pattern that defines how components obtain their dependencies. Instead of developing and maintaining dependencies within the component itself, this design provides them to the component.
4. MVC Architecture :
- Model – Represents the application data. Both business logic and data are stored and managed by it. Because of AngularJS’s two-way data binding, the View is automatically updated if the model changes.
- View – The user’s visible portion. i.e.., DOM
- Controller – Regular JS objects that control the data of AngularJS applications.
Example for AngularJS Module and Controller creation :
const app = angular.module('myApp', []); app.controller(‘exampleController’, function ($scope) { $scope.greetingText = ‘Hello, AngularJS!’; });
What is Angular?
Angular is a modern, component-based framework written in TypeScript. It was a complete rewrite of AngularJS, designed to address the shortcomings of its predecessor and building large-scale, complex applications for both web and mobile environments. With modularity, efficiency, and sophisticated tools, Angular offers a more structured approach than AngularJS.
Key Features of Angular :
1. TypeScript :
It is a superset to JavaScript(JS). It adds support for strict and strong typing to the JS syntax. It saves the user time by enforcing types and preventing unexpected value type changes prior to compilation.
2. Component-based Architecture :
This feature facilitates application maintenance while encouraging modularity and reusability. Each component consists of:
- Metadata (decorators) – Defines the component interaction with the application.
- Class (TypeScript code) – This holds actual business logic.
- View – Nothing but HTML template.
3. Strucutre of an Angular component:
@Component({ selector: 'app-example', templateUrl: './example.component.html', styleUrls: ['./example.component.css'] }) export class ExampleComponent { title = 'Hello Angular'; }
4. Data Binding :
This concept links an application’s User Interface (UI) and the data tat is shown there. To facilitate communication between components and templates, Angular provides a variety of data binding mechanisms.
Types of Data Binding in Angular :
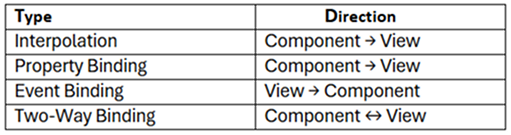
Let us explore each type in detail,
i. Interpolation {{ }} : Used to attach information to the template from the component class. It employs the syntax {{}}.
<h1> {{userName}} </h1>
Here, userName is a variable in the component, and its value will be displayed in the <h1> tag.
ii. Property Binding [ ] : Used to bind the value of a component property to an HTML element property. It uses [] (square brackets) around the HTML element property.
<img [src]="imageUrl" alt="logo" />
Here, imageUrl is bound to src property of the <img /> tag.
iii. Event Binding ( ) : Used to bind events raised in the view (like click) to methods in the component. It uses () around the event.
<a class="link" (click)="generateTemplate()">Generate Template</a>
Here, click event is bound to the generateTemplate method in the component.
iv. Two-way Data Binding [(ngModel)] : It makes data to be available in component and template i.e.., changes in the view are reflected in the component and vice versa. It uses a combination of property binding and event binding.
<textarea [(ngModel)]="comments" rows="14"></textarea>
Here, Comments is bound to the textarea element. Any changes in the textarea will update the comments property in the component, and vice versa.
5. Forms :
Forms are an essential part of web applications, enabling user input collection, validation, and submission.
Angular supports two types of forms :
Each approach has distinct advantages, and choosing between them depends on the complexity and requirements of the application.
- Template-driven forms –Template-driven forms are suitable for simple and static forms where minimal logic is required. They rely on Angular’s directives (ngModel, ngForm) to manage form validation and data binding. The HTML template is where the form structure and validation are completed.
Key concepts include:
i. NgModel – Uses two-way data binding to connect data between the component class and the form.
ii. FormModule – This needs to be imported into the module that uses the form.
- Reactive forms – Reactive forms, also known as model-driven forms, are ideal for complex situations where forms must be dynamic and programmatically managed since they provide you more control over the form logic and validation.
Key Concepts include:
i. Form Control : It is responsible for monitoring the value and validation status of a single form input.
const formControl= new FormControl(' ', Validators.required);
ii. Form Group : It is made up of several Form Control objects that monitor the total value and validation state of the form as a whole.
const formGroup= new FormGroup({ 'user ID' : new FormControl( ' ' ), 'password': new FormControl( ' ' ) });
iii. Form Array : It is a collection of instances of Form Group or Form Control, helpful for generating a dynamic control list.
const formArray= new FormArray([ new FormControl( ' ' ), new FormControl( ' ' ) ]);
Differences between Template-driven and Reactive Forms
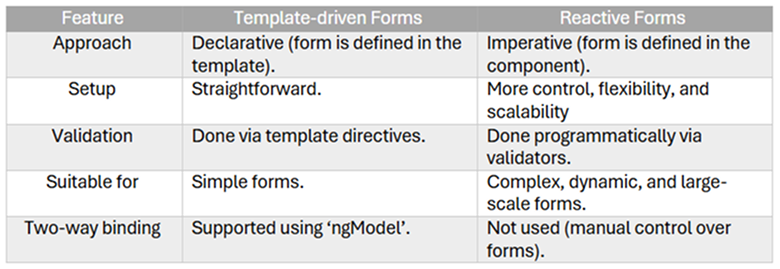
Component Lifecycle stages
From creation to destruction, each component has a certain lifecycle. These changes include :
- Creation (Initialization).
- Change Detection.
- Destruction (Clean up).
Lifecycle Hooks :
Each lifecycle hook plays a crucial role in the initialization, updating, and destruction of an Angular Component. Below is the practical representation of Angular life cycle hooks. For a detailed explanation, we can always refer to the Angular documentation.
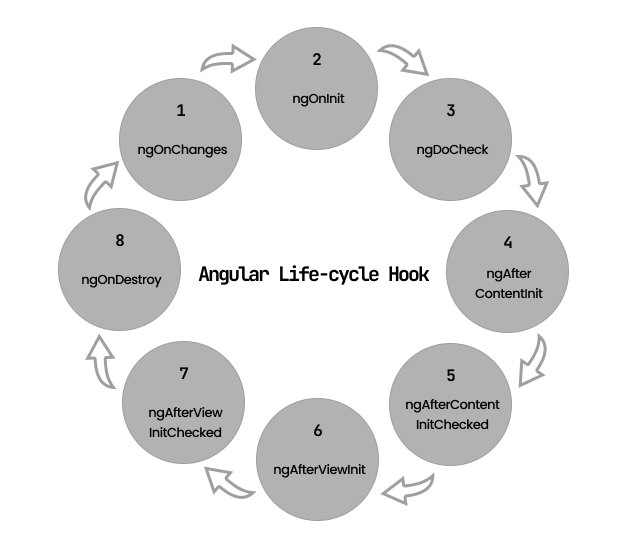
Example for Angular Component code :
@Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.scss'] }) export class AppComponent{ userName: string= 'Hello World!'; }
AngularJS vs Angular
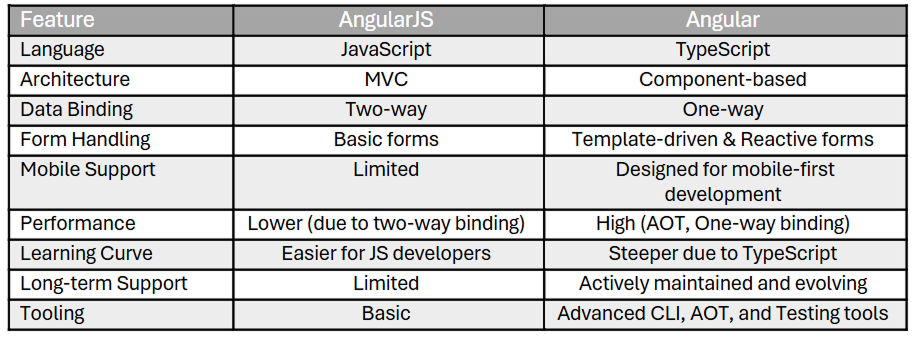
When to Use AngularJS vs Angular?
Use AngularJS When :
- Working on legacy projects that already use AngularJS.
- Developing small-scale applications with basic features.
- The team is proficient in JavaScript but not TypeScript.
Use Angular When :
- Building modern, scalable, and high-performance applications.
- Developing mobile-first and cross-platform applications.
- More suited for large applications requiring advanced tooling and performance optimization.
Disadvantages of AngularJS and Angular
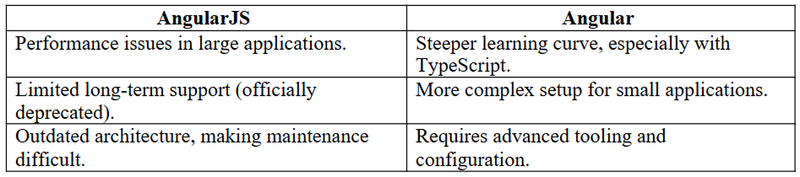
Conclusion :
Both AngularJS and Angular are powerful tools, but they serve different purposes:
- AngularJS is suitable for small, legacy projects that do not require high scalability.
- Angular is ideal for modern, scalable, and mobile-friendly applications due to its performance, modularity, and long-term support.
For new projects, Angular is the recommended choice due to its better architecture, enhanced tooling, and support for modern web development standards.